Photo Face Swap Google Colab
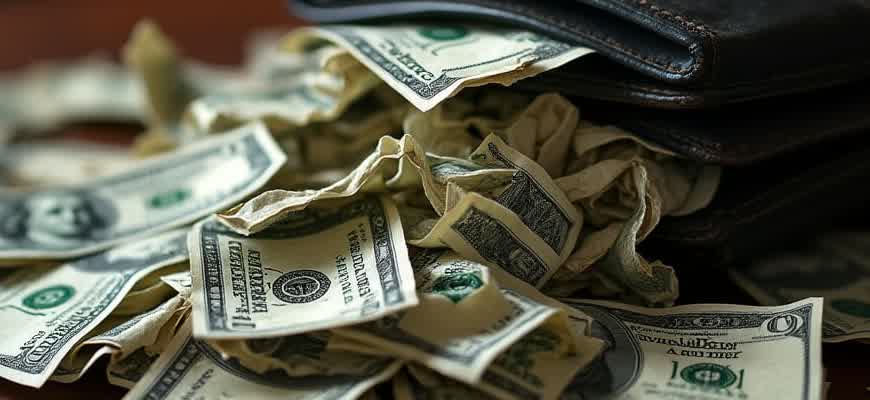
Face swapping is a popular technique in image processing where the facial features of two people are exchanged. Google Colab, a cloud-based Python environment, provides an easy and efficient platform for running face swap algorithms. The key advantage of using Google Colab for this task is its access to powerful GPUs, which speeds up the process significantly.
To perform a face swap in Google Colab, users typically follow a series of steps:
- Upload source images to Google Colab environment.
- Install necessary Python libraries, such as OpenCV and dlib, for image manipulation and face detection.
- Detect facial landmarks using pre-trained models to ensure accurate alignment of faces.
- Swap the faces while preserving the original image quality and lighting conditions.
Important: Ensure that you are using images with similar angles and lighting for best results in face swapping.
The overall process requires several key components:
Component | Description |
---|---|
Image Preprocessing | Resizing and aligning the faces before performing the swap. |
Face Detection | Using models like OpenCV or dlib to locate facial features. |
Face Replacement | Swapping the facial regions while adjusting for color and texture matching. |
How to Set Up Face Swap on Google Colab
Face swapping is a popular technique for manipulating images, where the faces of two or more people in a photo are exchanged. Using Google Colab for this task is a great way to leverage its cloud computing capabilities without worrying about local hardware limitations. Google Colab offers an easy-to-use platform that supports Python libraries and allows seamless integration with machine learning models for photo manipulation, including face-swapping. In this guide, we will go over the steps to set up face swapping using Colab and relevant tools.
Setting up a photo face swap on Google Colab involves installing necessary libraries, uploading images, and running pre-trained models. By following these steps, you will be able to swap faces in images in just a few minutes. Below are the key steps and components needed to complete this task:
Steps for Setting Up Face Swap
- Step 1: Set up the environment
- Open a new notebook in Google Colab.
- Install required libraries, such as
opencv-python
,dlib
, andnumpy
. - Import necessary modules for image processing and model loading.
- Step 2: Upload your images
- Upload two images containing the faces you want to swap.
- You can upload images directly from your computer or use images from a URL.
- Step 3: Use the pre-trained model
- Load a face detection model (e.g.,
MediaPipe
ordlib
) to detect faces in the images. - Apply the face swap model, which will swap the faces of the detected faces between the two images.
- Load a face detection model (e.g.,
- Step 4: Display the result
- Once the faces are swapped, use
Matplotlib
to display the output.
- Once the faces are swapped, use
Important Notes
Make sure your image sizes are similar, as large disparities in image resolutions might result in errors during the face swap process.
Once everything is set up, you will be able to run the code and see the swapped faces in real time. Below is an example of how the image processing flow can look in a table:
Step | Action |
---|---|
1 | Install libraries like OpenCV and dlib |
2 | Upload images to Colab environment |
3 | Detect faces using machine learning model |
4 | Perform face swapping using pre-trained model |
5 | Display the output image with swapped faces |
Step-by-Step Instructions to Upload Your Images for Face Swapping
Uploading images for face swapping in Google Colab requires careful preparation. To start, ensure your photos are in the correct format, usually JPEG or PNG, and the faces are clearly visible in both images. Once ready, follow the steps below to upload your files into the Google Colab environment.
Follow these detailed instructions to seamlessly upload your images for the face-swapping process:
- Prepare Your Images: Ensure that both the source and target images are in an appropriate format and of good resolution. Photos with clear faces work best.
- Open Google Colab: Navigate to the Google Colab website and create a new notebook for your project.
- Mount Google Drive: Mount your Google Drive to access and save files. Run the following command:
from google.colab import drive drive.mount('/content/drive')
- Upload Images: Use the Colab interface to upload your source and target images manually or from your Google Drive. You can upload files with the following code:
from google.colab import files uploaded = files.upload()
- Verify Upload: Once the files are uploaded, use the command below to check if the files are available:
import os os.listdir()
Note: The image files should appear in the working directory after the upload process. Ensure that both images are accessible before proceeding with the face swap.
File Management in Google Colab
After uploading the images, you may need to organize your files for easier reference. Here’s a simple way to manage the files in the Google Drive environment:
File Name | Location |
---|---|
Source Image | /content/drive/MyDrive/source_image.jpg |
Target Image | /content/drive/MyDrive/target_image.jpg |
Remember to keep your images in an easily accessible location within your Drive to simplify the face-swapping process.
Choosing the Right Model for Accurate Face Swapping
When performing face swapping tasks, selecting the appropriate model is crucial for obtaining high-quality results. The choice of the model significantly affects how well facial features are swapped while maintaining the realism of the output. A model must be capable of handling challenges such as varying lighting conditions, face angles, and different facial expressions. The goal is to ensure that the swapped face blends seamlessly with the background and the other person's features.
In the context of face-swapping technology, not all models are created equal. Some are designed to preserve the texture and natural appearance of the skin, while others may focus more on the accurate positioning and alignment of facial features. Understanding the specific strengths and weaknesses of each model is key to achieving optimal results.
Factors to Consider in Model Selection
- Accuracy of Facial Landmarks: The precision with which a model identifies and maps key facial features (eyes, nose, mouth, etc.) is critical for achieving a convincing face swap.
- Realism of the Output: Some models are better at maintaining natural skin tones and lighting conditions, which is essential for seamless integration between the swapped face and the target image.
- Performance and Speed: For large datasets or real-time applications, the efficiency of the model becomes important. Models that are optimized for speed can be more suitable for practical use cases.
Popular Models for Face Swapping
- DeepFaceLab: A popular deep learning-based model known for its flexibility and high-quality results, especially for high-resolution images. It supports both face replacement and face aging techniques.
- Faceswap: An open-source project that utilizes machine learning for face swapping and is favored for its ease of use and customizable features.
- StyleGAN2: A generative adversarial network (GAN) that can be adapted for face swapping, especially effective in generating photorealistic faces.
Comparison Table
Model | Realism | Speed | Customizability |
---|---|---|---|
DeepFaceLab | High | Medium | High |
Faceswap | Medium | High | Medium |
StyleGAN2 | Very High | Low | Low |
Choosing the right model depends on the project needs–whether you prioritize realism, speed, or customizability. It’s important to test different models and configurations to find the one that best suits your specific requirements.
How to Resolve Face Detection Issues in Google Colab
Face detection errors can arise due to various reasons when working with photo manipulation tasks in Google Colab. These issues may range from improper alignment to failure in detecting faces altogether. Understanding the common causes and how to resolve them will help streamline the face swap process. Here, we will outline effective strategies for managing such errors.
In general, problems with face detection can be categorized into issues with the input image, incorrect model configurations, or limitations of the face detection algorithm. Resolving these errors often requires adjusting the input data or tweaking the environment settings in Google Colab.
Common Face Detection Problems and Solutions
- Low-Quality Input Image: Low-resolution images or poor lighting can make it difficult for the model to detect faces accurately. Ensure high-quality, well-lit images with clear facial features.
- Incorrect Image Format: Some face detection algorithms may not support certain image formats. Convert your images to a widely accepted format such as JPG or PNG.
- Incorrect Model Configuration: The default parameters of the detection model may need adjustment for different types of images or faces. You may need to fine-tune the model's settings based on your dataset.
Step-by-Step Error Handling
- Check Input Data: Make sure the input images are of sufficient quality and resolution. Also, ensure that the image is properly pre-processed (e.g., resizing, normalization).
- Use a Robust Model: Some face detection algorithms work better in challenging conditions. Switch to a more advanced model like MTCNN or Dlib if the default method fails.
- Examine Logs: Review any error messages or logs generated during the face detection process to pinpoint the root cause of the issue.
Tip: Always validate that your image contains clear, unobstructed faces for better detection performance.
Common Face Detection Models in Google Colab
Model | Advantages | Limitations |
---|---|---|
MTCNN | Good at detecting faces in different orientations and lighting | Slower performance on large datasets |
Dlib | Highly accurate in detecting faces | Can be computationally expensive |
OpenCV Haar Cascade | Fast and lightweight | Less accurate, especially with faces in non-frontal poses |
Optimizing Image Quality for Realistic Face Swaps
Achieving high-quality and realistic face swaps involves careful attention to various factors that influence image quality. To ensure a seamless integration of faces, several key elements must be considered, such as lighting consistency, facial alignment, and texture matching. These aspects help in maintaining the natural look of the final composite, avoiding distorted features or unnatural transitions between the two faces.
The process of optimizing the image quality also requires a deep understanding of the underlying algorithms used for facial recognition and blending. Fine-tuning these algorithms allows for more accurate facial feature detection and a better overall match in terms of skin tone, lighting, and facial expression. In this section, we will explore the essential steps for improving the realism of face swapping projects in Google Colab environments.
Key Steps for Enhancing Face Swap Realism
- Facial Landmark Detection: Accurate alignment of facial features is critical. Detecting and matching landmarks such as eyes, nose, and mouth ensures proper placement.
- Color and Lighting Adjustment: Matching the skin tone and lighting conditions between the source and target faces helps to blend them together seamlessly.
- Texture Mapping: Ensuring that textures like skin, wrinkles, and other facial features are transferred properly can significantly improve realism.
Techniques for Image Refinement
- Image Resolution Scaling: Higher resolution images provide more detail, which is essential for maintaining clarity during the blending process.
- Deep Learning Models: Using advanced deep learning algorithms, such as GANs (Generative Adversarial Networks), can improve the quality of facial swaps by learning from large datasets.
- Post-Processing Filters: After the swap, applying smoothing or sharpening filters can enhance the final image and remove any visible artifacts.
Important Tip: Always ensure that the source and target images have similar head poses and angles to avoid mismatched perspectives, which can make the swap look unnatural.
Impact of Algorithm Parameters on Face Swap Quality
Parameter | Effect on Quality |
---|---|
Learning Rate | Adjusting the learning rate of the model can help in fine-tuning the results and avoiding overfitting or underfitting. |
Image Augmentation | Using techniques like rotation, scaling, and flipping can increase dataset diversity, leading to better generalization in face swaps. |
Understanding the Limitations of Face Swapping on Google Colab
Although face swapping using Google Colab is an exciting application of deep learning and computer vision, it is important to understand its inherent limitations. While the platform provides access to powerful resources like GPUs and pre-trained models, there are specific challenges that can affect the quality and effectiveness of face-swapping processes. These limitations stem from the nature of the model, computational constraints, and potential ethical concerns when dealing with sensitive images.
In order to get the best results, users need to be aware of certain factors that can hinder the process. This includes everything from the accuracy of face alignment to the hardware restrictions inherent in Google Colab's free-tier environment. Below are some of the key limitations when using Google Colab for face-swapping tasks:
- Model Performance: Pre-trained models may not always work well for all types of faces or images.
- Quality of Output: The final image can be distorted or have visible artifacts, especially when the source and target faces differ in size or angle.
- Computational Constraints: Google Colab's free-tier GPU may be insufficient for complex, high-resolution face swaps.
- Privacy Concerns: Using personal or sensitive images in an online environment raises potential privacy issues.
Key Factors Affecting Face Swap Quality
- Face Alignment: Accurate face positioning is critical. Misalignment can lead to distorted facial features in the final image.
- Resolution Limits: Google Colab imposes resolution constraints on images that can result in lower-quality swaps.
- Limited Resources: The free-tier Colab instance can be slower, affecting the speed and output quality of face swaps.
It is essential to test the model on various types of faces to understand its limitations before deploying it for a larger project or sensitive tasks.
Table: Comparison of Google Colab Free and Pro Versions
Feature | Free Version | Pro Version |
---|---|---|
GPU Availability | Limited access | Priority access |
Memory | 12GB | 25GB |
Runtime Duration | Up to 12 hours | Up to 24 hours |
Cost | Free | $9.99/month |
How to Download and Save Your Face Swap Results
After completing a face swap process in Google Colab, saving the final images is a crucial step to preserve your work. Whether you plan to share the results or simply store them for future reference, there are multiple methods to download and save your face-swapped images efficiently.
Google Colab provides a few straightforward techniques to download your results directly to your local system. Below is a guide on how to do this without any complications.
Method 1: Using Colab's File System
Google Colab offers a simple way to save images using its built-in file system. The images can be stored in the Colab environment and then downloaded to your local machine.
- Once the face swap process is complete, ensure that the final image is stored in the correct directory within the Colab file system.
- Use the following code to download the image directly:
from google.colab import files files.download('/content/your_image.jpg')
- Click the link that appears after running the code, and the image will be saved to your computer.
Method 2: Save Images to Google Drive
If you'd prefer to save your swapped faces to Google Drive for easier access and sharing, you can mount Google Drive in your Colab session. This method offers the benefit of cloud storage, keeping your images safe and accessible from anywhere.
- First, mount your Google Drive with the following command:
from google.colab import drive drive.mount('/content/drive')
- After mounting, navigate to the folder in your Drive where you'd like to save the image.
- Move the image to the folder with:
!cp /content/your_image.jpg /content/drive/MyDrive/your_folder/
- Access your Google Drive folder to view or download the image from any device.
Important Notes
Ensure that the image path is correctly specified, especially when using the `files.download()` method, as incorrect paths can lead to failed downloads.
Summary
Both of these methods provide reliable ways to save and download your face swap results. The first method is ideal for quick downloads directly to your device, while the second offers the advantage of cloud storage for easy access and sharing.
Integrating Face Swap with Python Libraries for Enhanced Image Editing
Face swapping is just one of many techniques used for image manipulation in Python. When combined with other libraries, it can open the door to more complex and refined image editing workflows. By leveraging a variety of tools such as OpenCV, Dlib, and TensorFlow, developers can enhance face-swapping processes with features like facial recognition, alignment, and even deep learning-based adjustments. These libraries not only improve the accuracy of face placement but also enable advanced transformations like age progression, facial expression modification, and lighting adjustments.
The combination of face swap algorithms with image processing libraries creates a powerful toolkit for dynamic and creative editing. OpenCV is often used for basic transformations and alignment, while libraries like Dlib can help with detecting facial landmarks to ensure precise swapping. Additionally, neural network-based libraries such as TensorFlow or PyTorch enable further enhancement by incorporating generative models for realistic image alterations.
Key Python Libraries for Face Swap Integration
- OpenCV: A comprehensive library for image processing that handles basic face detection, manipulation, and alignment.
- Dlib: Known for its robust facial landmark detection, it helps with ensuring precise positioning of faces during swaps.
- TensorFlow / PyTorch: Deep learning frameworks that can be used to enhance face swap results, such as for generating realistic facial features or adjusting expressions.
- Face Recognition: A library built on Dlib, it simplifies the process of facial recognition and alignment, crucial for successful swaps.
Advanced Editing Techniques Using Integrated Libraries
- Facial Alignment: Ensuring the faces align properly using Dlib’s facial landmark detection.
- Expression Modulation: Applying neural networks to modify facial expressions before or after swapping.
- Lighting Adjustments: Enhancing lighting conditions in face-swapped images using OpenCV’s color manipulation functions.
- Age and Gender Transformation: Using generative models to simulate age progression or change in gender representation.
Important Note: When integrating these libraries, ensure that proper error handling and optimization techniques are applied to prevent performance bottlenecks during the image processing steps, especially when dealing with high-resolution images.
Example Workflow
Step | Library | Task |
---|---|---|
Step 1 | OpenCV | Detect and align faces in the source and target images. |
Step 2 | Dlib | Extract facial landmarks for precise placement of the face. |
Step 3 | TensorFlow / PyTorch | Refine facial features and generate realistic adjustments. |
Step 4 | OpenCV | Final touch-ups such as lighting correction and blending. |