Deepfake Face Swap Python
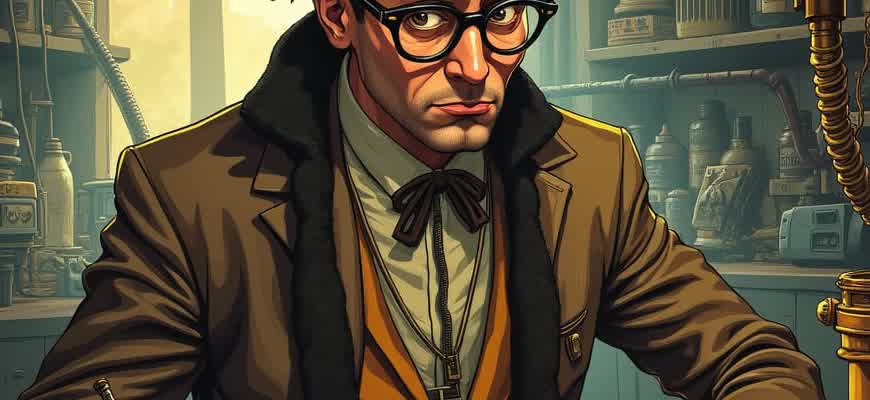
Replacing one person's facial features with another in video content has become increasingly accessible thanks to open-source Python libraries. This technology, built on deep neural networks, enables frame-by-frame identity transfer by combining face detection, alignment, and generative modeling.
- Face Detection: Locates facial regions using models like MTCNN or RetinaFace.
- Landmark Alignment: Normalizes facial geometry using 68-point landmark models for consistent input.
- Latent Encoding: Encodes the source and target face into latent vectors with autoencoders or GANs.
Note: High-quality swaps require consistent lighting, angle matching, and extensive training data for the generator model.
Common Python libraries for facial identity synthesis include:
- FaceSwap: Modular project with support for training and real-time swapping.
- DeepFaceLab: High-accuracy toolkit with custom model architectures.
- InsightFace: Face recognition and alignment tools integrated into swap pipelines.
Library | Main Feature | Typical Use Case |
---|---|---|
FaceSwap | Real-time preview, plugin system | Interactive face replacement projects |
DeepFaceLab | High-fidelity output, model variety | Film-level face editing |
InsightFace | State-of-the-art face recognition | Embedding-based identity transfer |
How to Install and Configure Libraries for Facial Identity Replacement in Python
To begin working with facial identity swapping in Python, you need to set up your development environment with the appropriate libraries and dependencies. The most common frameworks include DeepFaceLab, FaceSwap, and Avatarify, each of which has specific requirements regarding system configuration and GPU support. It is essential to ensure compatibility with CUDA-enabled GPUs for accelerated performance during model training and inference.
Python versioning and virtual environments play a key role in managing dependencies. Tools like virtualenv or conda help maintain isolated environments, preventing package conflicts. You’ll also need to install key Python packages such as dlib
, opencv-python
, and tensorflow
or torch
, depending on the framework you choose.
Step-by-Step Configuration Guide
- Create a virtual environment:
- Using virtualenv:
virtualenv faceswap_env
- Using conda:
conda create -n faceswap_env python=3.8
- Using virtualenv:
- Activate the environment:
source faceswap_env/bin/activate
(Linux/macOS)faceswap_env\Scripts\activate
(Windows)
- Install core dependencies:
pip install numpy opencv-python dlib
pip install tensorflow
orpip install torch torchvision
- Clone and install a library repository (e.g., FaceSwap):
git clone https://github.com/deepfakes/faceswap.git
cd faceswap
python setup.py install
Note: CUDA and cuDNN must be installed and match the version of TensorFlow or PyTorch. Mismatches may cause runtime failures or silent performance degradation.
Library | Python Version | GPU Support | Installation Method |
---|---|---|---|
DeepFaceLab | 3.6 - 3.8 | CUDA 11+ | Precompiled binaries / source |
FaceSwap | 3.8 | Optional | Git + pip |
Avatarify | 3.7 | Required | Docker / source |
Choosing Between DeepFaceLab, Faceswap, and Custom Models
When working with facial replacement in Python, the choice of tool significantly impacts both the quality of results and the workflow complexity. Three primary options dominate the space: DeepFaceLab, Faceswap, and building custom pipelines with frameworks like PyTorch or TensorFlow. Each has distinct advantages depending on your goals, whether you're optimizing for realism, training speed, or flexibility.
DeepFaceLab offers a tightly optimized pipeline for high-resolution face swapping. It's favored for producing professional-grade output, especially for video. Faceswap, by contrast, provides a more user-friendly interface and modular design, making it easier to experiment with different models and preprocessing techniques. A custom setup, while requiring more initial development, allows full control over architecture, data augmentation, and training loops.
Comparison Overview
Feature | DeepFaceLab | Faceswap | Custom Models |
---|---|---|---|
Ease of Use | Low | Moderate | Very Low |
Output Quality | High | Moderate | Variable |
Customization | Limited | Medium | Full |
Community Support | Large | Moderate | Small |
Note: If you aim for maximum visual fidelity in video production, DeepFaceLab remains the preferred choice. For prototyping or learning, Faceswap's interface and plugin system are more approachable.
- DeepFaceLab: Best for advanced users focused on production-quality output.
- Faceswap: A balanced choice for experimentation and educational use.
- Custom Solutions: Ideal for research or integrating novel architectures.
- Assess your technical proficiency and time budget.
- Determine whether output quality or flexibility is more critical.
- Choose a tool aligned with your project's scale and goals.
Preparing Training Data: Facial Alignment and Data Cleaning
High-quality synthetic face-swapping models rely heavily on the precision of input data. Before training begins, each image must undergo geometric normalization–ensuring that facial landmarks such as eyes, nose, and mouth are consistently aligned across the dataset. This eliminates unnecessary variability and allows the neural network to focus on actual facial features instead of compensating for misalignment.
Equally important is purging the dataset of noisy or corrupted samples. Low-resolution images, motion blur, incorrect face crops, and extreme lighting conditions degrade model accuracy. Ensuring clean, consistent input is the foundation for generating realistic and coherent face-swapped outputs.
Facial Normalization Pipeline
- Detect key facial landmarks using models like MTCNN or dlib's shape predictor.
- Apply affine transformations to rotate, scale, and translate faces into a canonical pose.
- Crop aligned faces to a fixed size, typically 256x256 or 512x512 pixels.
Note: Misaligned faces lead to training artifacts, such as warping and inconsistent lighting. Alignment must be exact for all samples.
Cleaning and Filtering the Dataset
- Manually inspect a subset of images to define quality thresholds.
- Remove samples with occlusions (e.g., hands, sunglasses) or poor illumination.
- Use automatic blur detection (e.g., Laplacian variance) to discard out-of-focus frames.
Issue | Impact | Recommended Action |
---|---|---|
Motion Blur | Loss of detail, feature smearing | Exclude frames with low sharpness |
Profile Faces | Model confusion due to missing features | Filter or augment with frontal faces |
Expression Variability | Inconsistent training results | Balance dataset across expressions |
Training a Custom Neural Model for Facial Identity Replacement
To build a model capable of swapping faces with high fidelity, it is essential to gather and preprocess a dataset specific to the source and target individuals. This involves extracting frames from videos, detecting and aligning faces, and organizing them into input-output pairs for supervised learning. The quality and quantity of this data directly impact the realism and consistency of the final results.
Once the dataset is ready, model training requires selecting an appropriate architecture, often based on autoencoders with shared encoders and dual decoders. Training typically proceeds over tens of thousands of iterations on a GPU, with periodic evaluations on validation images to monitor identity preservation and artifact minimization.
Steps for Preparing Your Dataset and Starting Training
- Video Frame Extraction: Use tools like FFmpeg to extract frames at 1-2 FPS to reduce redundancy.
- Face Detection & Alignment: Apply MTCNN or dlib to crop and align faces to a consistent size (e.g., 256x256 pixels).
- Dataset Organization: Structure folders as Person_A and Person_B, ensuring balanced representation.
- Preprocess faces with normalization and optional augmentations (e.g., flips, lighting changes).
- Load data into the training pipeline using data generators or PyTorch DataLoaders.
- Train the model, saving checkpoints and loss metrics for analysis.
Tip: At least 500–1000 face images per person are recommended to achieve a stable model with minimal visual glitches.
Component | Tool/Method | Purpose |
---|---|---|
Frame Extraction | FFmpeg | Extract stills from video sources |
Face Detection | MTCNN / dlib | Locate and align facial regions |
Model Type | Autoencoder | Encode shared features, decode per identity |
Real-Time Facial Overlay with Python and OpenCV
Implementing dynamic facial overlays in live video streams involves tracking key facial landmarks and accurately mapping one face onto another in motion. Using Python in combination with OpenCV, it becomes possible to capture real-time webcam input, detect facial geometry, and blend another face frame-by-frame while maintaining natural head movements and expressions.
This method primarily relies on facial landmark detection (typically using Dlib or Mediapipe), affine transformations for warping, and seamless cloning for blending. The process ensures that the swapped face fits perfectly within the contours and lighting of the target face, creating a smooth and believable overlay.
Core Steps of the Implementation
- Capture live video frames using cv2.VideoCapture().
- Detect facial features using a landmark predictor.
- Extract and align facial regions using affine transformations.
- Overlay the new face onto the source frame.
- Blend edges with seamless cloning to eliminate sharp transitions.
Note: Using lightweight models like Mediapipe enables high-speed tracking on standard CPUs, which is crucial for real-time applications.
- Facial tracking must be robust to rotation and lighting changes.
- Precision in landmark detection directly affects overlay quality.
- Latency must be minimal for a smooth user experience.
Component | Description |
---|---|
Landmark Detection | Identifies key facial points (eyes, nose, jawline). |
Affine Transformation | Warps one facial region to match the target's geometry. |
Seamless Cloning | Blends the swapped face to match lighting and texture. |
Integrating Synthesized Face Replacements into Post-Production Pipelines
When working with AI-driven facial identity replacement, embedding the generated output into a professional video editing environment demands precise asset management and seamless synchronization. The modified frames or video segments must align perfectly with the original timeline, audio tracks, and visual effects layers. Frame-accurate exports with alpha channels are often essential to retain flexibility in compositing.
Effective integration begins with rendering deep learning outputs into formats compatible with non-linear editors like Adobe Premiere Pro, DaVinci Resolve, or Final Cut Pro. This typically involves exporting the altered footage as image sequences (e.g., PNG with transparency) or high-bitrate video files (e.g., ProRes or DNxHR) for lossless quality retention during the color grading and final delivery stages.
Key Steps for Post-Production Integration
- Align the synthetic frames with the original video’s frame rate and resolution.
- Use timecode references to synchronize the AI-generated sequences with the base timeline.
- Apply color matching to maintain visual consistency between original and altered clips.
- Overlay with original scene lighting using blending modes or LUTs for realism.
- Prefer image sequences for per-frame adjustments.
- Use proxy editing if the generated footage is high-resolution.
- Maintain original audio untouched to avoid desynchronization artifacts.
Output Type | Recommended Format | Use Case |
---|---|---|
Short clip swap | ProRes 4444 | Quick insert into existing edit |
Full-scene modification | PNG Sequence | Precise frame-level compositing |
Social media edit | MP4 (H.264) | Fast export with smaller size |
For maximum flexibility, always retain uncompressed versions of AI-generated visuals before merging into final edit timelines.
Optimizing GPU Performance for Faster Model Inference
Optimizing the use of GPU resources is crucial for enhancing the efficiency of deepfake models, especially during face-swapping tasks. Deep learning models, particularly those involved in image manipulation, require substantial computational power. By leveraging GPU capabilities efficiently, inference times can be significantly reduced, which is essential for real-time applications.
Proper GPU optimization involves understanding how different components of the model interact with the hardware. This includes optimizing memory usage, adjusting batch sizes, and leveraging parallel processing techniques. Below are practical strategies to enhance GPU performance during model inference.
Key Techniques for GPU Optimization
- Memory Management: Efficient memory usage is essential for optimal performance. Utilizing memory pooling, reducing the model size, or using tensor decompositions can minimize memory overhead.
- Batch Size Adjustment: Finding the right batch size is crucial. Too large can lead to out-of-memory errors, while too small can underutilize GPU capacity.
- Mixed Precision Training: Using lower precision (such as FP16 instead of FP32) can speed up computation without significantly compromising accuracy.
- Parallelization: Leveraging multi-GPU setups or parallel execution across available cores can drastically speed up inference times for larger models.
Performance Gains with Parallelization
- Data Parallelism: Distributes the data across multiple GPUs, allowing for concurrent processing of different data batches.
- Model Parallelism: Divides the model into smaller parts, enabling each GPU to work on different sections of the model simultaneously.
- Pipeline Parallelism: Stages the model computation across GPUs, where each GPU processes different phases of the model's computation.
Example Performance Comparison
GPU Configuration | Inference Time (Seconds) | Memory Utilization (%) |
---|---|---|
Single GPU | 12.5 | 85 |
Dual GPU | 7.8 | 90 |
Quad GPU | 4.3 | 95 |
By utilizing advanced memory management and parallelization techniques, deepfake models can process images significantly faster, allowing for smoother, real-time performance in face-swapping applications.
Improving Visual Integrity and Eliminating Artifacts in Face Swaps
Detecting and eliminating artifacts is crucial in achieving high-quality face swaps, particularly when it comes to deepfake technology. These imperfections can detract from the realism of a swapped face, making it easier to detect manipulation. To improve the visual consistency, both the source and target faces must blend seamlessly, avoiding visible seams or discrepancies in texture, lighting, and alignment.
Several methods can be applied to improve the overall appearance of swapped faces. The primary focus is on enhancing the blending of facial features and minimizing the visible differences that arise from inconsistent lighting, skin tones, or facial shapes. A combination of pre-processing techniques and post-processing corrections is often required to achieve optimal results.
Strategies for Detecting and Fixing Artifacts
- Lighting and Color Matching: Ensure that the lighting conditions of both the source and target faces match to avoid unnatural brightness or shadows.
- Edge Blending: Seamlessly merge the edges of the swapped face with the background to reduce visible lines or sharp transitions.
- Texture Adjustment: Use tools to fine-tune the texture of the face to match the surrounding skin tone and detail.
- Facial Feature Alignment: Ensure the key facial features, such as eyes, nose, and mouth, are perfectly aligned with the target face to prevent awkward positioning.
Common Artifacts in Face Swaps
- Blurry Edges: Occurs when the edges of the swapped face do not align well with the target, causing an unnatural transition between the face and the background.
- Inconsistent Lighting: When the lighting on the swapped face does not match the environment, it leads to a jarring, unrealistic appearance.
- Color Mismatches: Differences in skin tone or texture between the source and target faces often cause an unnatural look.
"The key to improving the realism of deepfake swaps lies in meticulously addressing lighting, texture, and alignment to ensure seamless integration with the target face."
Advanced Techniques for Enhancing Face Swap Quality
Technique | Description |
---|---|
Generative Models | Advanced neural networks can learn from large datasets to generate more realistic face swaps with fewer artifacts. |
Multi-Scale Refinement | Refining the swapped face in multiple scales ensures finer details are aligned and corrected, reducing visible artifacts. |
Post-Processing Filters | Applying filters to smooth out discrepancies in color and texture helps improve visual consistency and reduce noticeable errors. |