R Create Factor Variable
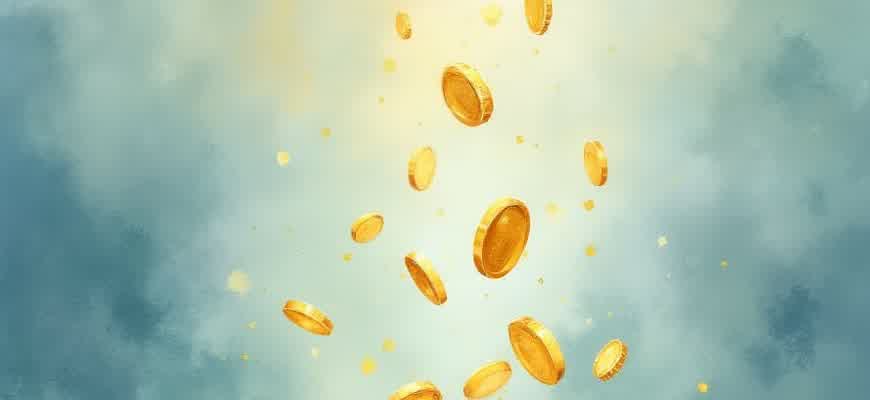
In R, a factor is an R data type used for categorical data. Factors are useful for representing variables that have a fixed number of unique values, such as "Male" and "Female" for gender or "Low", "Medium", and "High" for risk levels. This data type is essential when working with statistical models and performing data analysis in R.
To create a factor variable in R, you can use the factor() function. The basic syntax of this function is as follows:
factor(x, levels = NULL, labels = NULL, ordered = FALSE)
- x: The vector or data to be converted into a factor.
- levels: The unique values that the factor can take.
- labels: Custom labels for each level.
- ordered: Specifies if the factor should be treated as an ordered factor.
The following table shows how factors are handled in R:
Original Data | Factor Representation |
---|---|
Low, Medium, High | Factor with levels "Low", "Medium", "High" |
Male, Female | Factor with levels "Male", "Female" |
Note: Factors are important in statistical modeling in R, as they indicate that a variable has a limited set of values, which helps R handle them efficiently in analyses such as regression or ANOVA.
How to Create Factor Variables in R for Better Data Analysis
In R, factor variables play a crucial role in handling categorical data effectively. When working with datasets that contain qualitative values (such as gender, region, or product type), it is essential to convert these variables into factors to ensure proper statistical analysis and visualization. Factor variables allow R to treat data as discrete groups, enabling more accurate modeling and interpretation.
To create a factor variable in R, the most common approach is by using the `factor()` function. This function transforms a vector into a factor, which is particularly useful for variables that have a fixed number of unique values or levels. By converting a variable into a factor, R treats it as a categorical variable rather than a continuous one, optimizing the performance of statistical methods and visualizations.
Steps to Create Factor Variables in R
- Start by defining the variable you want to convert. For example, if you have a vector of data, you can directly apply the
factor()
function: - Check the levels of the factor to see how R has categorized the data:
- If necessary, you can set specific levels and labels for the factor by passing them as arguments:
gender <- c("Male", "Female", "Female", "Male")
gender_factor <- factor(gender)
levels(gender_factor)
gender_factor <- factor(gender, levels = c("Male", "Female"), labels = c("M", "F"))
Why Use Factor Variables in R?
- Efficient Memory Usage: Factor variables take up less memory compared to character vectors, especially when there are many repeated values.
- Better Data Handling: Factors help in grouping and categorizing data for more accurate statistical models and plots.
- Statistical Modeling: Many functions, such as regression models, require categorical variables to be factors to properly analyze them.
Converting a variable to a factor is essential when working with categorical data in R. It ensures correct interpretation by statistical models and optimizes computational efficiency.
Factor Example with a Data Frame
If you are working with a data frame, you can create a factor variable for any column that contains categorical data. For instance, consider the following table:
Person | Gender |
---|---|
Alice | Female |
Bob | Male |
Charlie | Male |
To convert the "Gender" column into a factor:
df <- data.frame(Person = c("Alice", "Bob", "Charlie"), Gender = c("Female", "Male", "Male"))
df$Gender <- factor(df$Gender)
Step-by-Step Guide to Assigning Levels in R Factor Variables
When working with categorical data in R, the factor variable type is commonly used to represent groups or categories. In R, factors come with a specific set of levels that define the possible values a factor can take. Assigning levels to a factor variable helps in controlling the order and interpretation of categories in your analysis, ensuring that your data is consistent and properly encoded.
This guide outlines the process of assigning and modifying levels in R's factor variables, allowing you to set up categorical data effectively for modeling or visualization purposes. By understanding how levels work, you can ensure that your analyses accurately reflect the intended structure of your data.
Assigning Levels Using `factor()`
To assign levels to a factor variable, you can use the `factor()` function. This function allows you to specify the order of levels, ensuring that R treats the levels in the sequence you intend.
- First, create a vector of categorical values:
data <- c("high", "medium", "low", "medium", "high")
- Next, convert this vector to a factor by specifying the desired levels:
factor_data <- factor(data, levels = c("low", "medium", "high"))
In this case, the levels are explicitly defined as "low", "medium", and "high". The factor variable will now respect this order when used in any analyses.
Modifying Existing Levels
If you have an existing factor and need to change the levels, you can use the `levels()` function. This is useful if you need to reorder or add new levels without re-creating the entire factor variable.
- To view the current levels of an existing factor:
levels(factor_data)
- To change the order of levels:
levels(factor_data) <- c("high", "medium", "low")
Note: Always ensure the correct order of levels when modifying factors, as it can influence the results of statistical tests and visualizations.
Table of Factor Levels Example
The following table illustrates the difference between factor levels before and after reordering:
Original Levels | Reordered Levels |
---|---|
low, medium, high | high, medium, low |
Understanding the Impact of Factor Variables on Data Visualization
Factor variables play a crucial role in the way categorical data is presented in visualizations. These variables allow data to be grouped and organized by categories, which aids in identifying trends, distributions, and patterns across different levels of data. Without converting variables into factors, the software might treat them as continuous data, leading to misinterpretation and misrepresentation of the information. Understanding how factor variables influence the presentation of data is essential for clear and accurate data visualization.
In data visualization, the correct handling of factor variables can significantly affect the clarity and comprehensiveness of your charts and graphs. These variables help categorize and display data in a way that makes it easier to compare different groups. By grouping data into categories (such as 'Male' and 'Female' or 'High', 'Medium', and 'Low'), factor variables allow you to generate meaningful visuals that are immediately interpretable. The impact of these variables extends to how visual tools like bar plots, boxplots, and histograms are structured.
How Factor Variables Affect Visual Representation
When factor variables are used in a plot, they impact several aspects of the data’s visual representation:
- Color Coding: Factors often drive the color schemes in visualizations, helping distinguish between categories visually.
- Axis Grouping: On plots like bar charts or scatter plots, factors are typically used to group data along categorical axes, making comparisons easier.
- Data Summarization: Factors are key in summarizing large datasets by breaking them into relevant categories, often used in boxplots and histograms.
Examples of Data Visualization Types Affected by Factor Variables
- Bar Charts: Display data grouped by factor levels, showing frequency distributions across categories.
- Boxplots: Use factor variables to compare distributions between different categories.
- Dot Plots: Highlight differences in distributions by placing points according to factor levels.
When factor variables are not correctly utilized, visualizations can become misleading, with data being treated as continuous, which may obscure key insights.
Example of Using Factor Variables in Data Visualization
Factor Category | Data Value | Visualization Impact |
---|---|---|
Gender | Male, Female | Used to differentiate data points by color or grouping in bar plots |
Age Group | Child, Adult, Senior | Segmented axis in histograms or boxplots to show variations across age categories |
Handling Missing Values in Factor Variables in R
Factor variables in R are commonly used to represent categorical data. However, like with any type of data, they may contain missing values, which can complicate analysis if not properly addressed. Missing data in factors may arise from various sources, such as errors during data collection or intentional omission. It is crucial to handle these missing values appropriately to ensure the integrity of statistical models and analyses.
In R, missing values in factors are typically represented by the NA value. Handling these missing values depends on the specific context and the nature of the dataset. Below are some strategies you can apply to manage missing values in factor variables:
Methods to Handle Missing Data
- Omission of Missing Values: One approach is to simply remove any rows that contain missing values in the factor variable. This method is useful when the missing values are minimal and their removal will not significantly impact the analysis.
- Imputation of Missing Values: Imputing missing values involves filling in missing data with a reasonable estimate. This can be done by assigning the most frequent category (mode) of the factor variable, or by using more complex imputation methods.
- Introduce a New Category: Another strategy is to create a new level within the factor to represent the missing data. This allows the missing data to be accounted for separately without affecting the rest of the analysis.
Code Example for Handling Missing Values in Factors
- Remove rows with missing values:
- Impute missing values with the mode:
- Create a new level for missing values:
data_clean <- na.omit(data)
mode_value <- names(sort(table(data$factor_variable), decreasing = TRUE))[1] data$factor_variable[is.na(data$factor_variable)] <- mode_value
data$factor_variable[is.na(data$factor_variable)] <- "Missing"
Important Considerations
Be cautious when choosing a method to handle missing values, as the approach you select can impact the outcomes of your analysis. Removing missing data can lead to a loss of valuable information, while imputation methods may introduce biases if not carefully applied.
Summary
Below is a summary table of the various methods to handle missing values in factor variables:
Method | Pros | Cons |
---|---|---|
Remove Rows | Simplicity, avoids bias in imputation | Loss of data, potential reduction in sample size |
Impute with Mode | Preserves sample size, easy to implement | Can introduce bias if distribution is skewed |
Create New Category | Retains all data, clear indication of missing values | May cause confusion, especially in analysis |
Converting Character Variables to Factors in R: A Practical Approach
In R, transforming character variables into factors is a common data preprocessing step. Factors are essential when working with categorical data, especially for statistical analysis and modeling. A factor in R is an R data type that stores categorical data and organizes it into levels, making it more efficient for analysis compared to character variables.
Converting character variables to factors can help streamline models and statistical tests, improving performance and accuracy. This is particularly useful when you want to represent categories such as gender, education level, or product type. Factors ensure R understands the variable as categorical, which is necessary for certain modeling algorithms and for creating efficient storage.
Steps to Convert Character Variables to Factors
- Step 1: Identify the character variables in your dataset.
- Step 2: Use the
factor()
function to convert the character variable into a factor. - Step 3: Verify the conversion and inspect the levels using the
levels()
function.
Example Conversion
- Assume you have a character variable called
Category
with values such as "Low", "Medium", and "High". - Convert it to a factor using the following code:
Category <- factor(Category)
. - Check the levels with
levels(Category)
to ensure proper conversion.
Important: Be aware that when you convert character variables to factors, R assigns default levels in alphabetical order unless specified otherwise.
Practical Example
Original Character Variable | Converted Factor Variable |
---|---|
Low, Medium, High, Low, High | Low, Medium, High |
As shown in the table, the character variable is converted into a factor with levels "Low", "Medium", and "High", which optimizes data handling in further analyses.
Best Practices for Using Categorical Variables in Statistical Models
Factor variables, or categorical variables, play a crucial role in statistical modeling, as they allow you to represent non-numeric data in a structured way. These variables can be used to capture groupings, classifications, or categories that are important for analysis. It’s essential to handle them properly to ensure that the resulting models are both accurate and interpretable.
To optimize the use of factor variables, consider the following guidelines. These practices help prevent misinterpretation, improve model performance, and make the process more efficient overall.
Key Guidelines for Working with Factor Variables
- Label Encoding vs. One-Hot Encoding: In many models, especially linear ones, the use of dummy variables (one-hot encoding) is standard. However, be cautious when using label encoding, as it may introduce ordinal relationships where none exist.
- Factor Levels: Ensure that the factor levels are ordered logically, especially in models that account for the order of categories (e.g., ordinal regression). Misordered levels may lead to erroneous interpretations.
- Handling Missing Data: Missing values in factor variables should be dealt with appropriately. Common strategies include treating missing categories as a separate level or using imputation methods.
- Regularization: When dealing with a large number of factor levels, regularization techniques like Lasso or Ridge regression can help to prevent overfitting.
Practical Considerations
- Factor Conversion: Always convert character variables to factors before incorporating them into models. In R, this can be done with the factor() function.
- Interpretation of Coefficients: When using factor variables in regression, the interpretation of coefficients refers to the difference between the reference category and other categories. Pay close attention to which category is set as the reference level.
- Interaction Effects: Don’t overlook potential interactions between factor variables. Including interaction terms can uncover hidden relationships between categorical variables that improve model accuracy.
Properly managing factor variables is essential not only for achieving accurate results but also for ensuring that your models are interpretable and meaningful.
Example: Factor Levels and Reference Category
Factor Level | Dummy Variable (One-Hot Encoding) |
---|---|
Low | 0 |
Medium | 1 |
High | 0 |
Optimizing Memory Usage with Factor Variables in R
R provides several ways to handle categorical data, and one of the most efficient methods is using factor variables. When working with large datasets, it is essential to manage memory usage efficiently. By converting character vectors to factors, significant memory savings can be achieved. This is particularly important when the dataset contains a large number of repeated categorical values, as it helps reduce the amount of memory consumed by the data.
Factors are stored as integers with corresponding levels. Each level is represented by an integer, and the character vector is replaced by a smaller integer vector. This reduces the memory footprint significantly, especially for columns with many repeated categories. Here, we’ll discuss key strategies to optimize memory usage in R when working with categorical data.
Memory Efficiency with Factors
One of the main benefits of using factors is that they store categorical variables more efficiently compared to character variables. The main difference lies in the way R stores the data:
- Character vectors: Each unique value is stored as a string, which can take up a lot of memory if there are many repeated categories.
- Factors: Store the unique values as levels and the data as integers, thus reducing memory usage.
Here’s a comparison of memory usage between character vectors and factors:
Data Type | Memory Usage |
---|---|
Character Vector | High (each string occupies memory space) |
Factor | Low (stored as integers with levels) |
Important: Converting a column to a factor can significantly reduce memory consumption, especially in data frames with many repeated categorical values.
How to Convert to Factors in R
Converting a variable to a factor is straightforward. The factor()
function in R helps transform a character vector or a numeric variable into a factor:
- Use
factor()
to convert the variable: - Use the argument
levels
to define the possible categories explicitly. - For large datasets, consider setting the argument
ordered = TRUE
if the categorical data has an inherent order (e.g., "low", "medium", "high").
Tip: Always check the structure of your data with
str()
to ensure that categorical variables are properly converted into factors.
Common Issues and Troubleshooting with Factor Variables in R
Factor variables in R are a powerful tool for managing categorical data, but working with them can sometimes lead to confusion and unexpected results. While factors are used for storing categorical data, they also come with a set of challenges that need to be addressed for effective data manipulation. Understanding these issues is key to improving the quality of your analyses.
One common issue is the mismanagement of factor levels, which can lead to errors when performing operations on the data. For example, when subsetting or reordering factors, you might unintentionally introduce discrepancies in the levels, resulting in incorrect analyses. In this guide, we will explore some common pitfalls and how to avoid them when working with factor variables.
Common Pitfalls in Factor Variables
- Factor Levels with Unexpected Order: One of the most common issues arises when the factor levels are not in the intended order. This can lead to incorrect results in plotting or statistical analysis where the levels' order matters.
- Introducing Unused Levels: When subsetting data, unused factor levels can remain in the factor variable, leading to confusion and unnecessary computations.
- Changing Levels After Creation: Modifying levels after a factor is created can sometimes result in errors or incorrect data mappings, especially when there is a mismatch between the original levels and the new ones.
Troubleshooting Factor Variables
- Ensure Correct Factor Level Order: When creating a factor, always specify the correct order of levels using the
levels
argument. This ensures that operations like sorting and plotting work as expected. - Remove Unused Levels: Use the
droplevels()
function to drop unused levels from a factor after subsetting the data. - Check Level Consistency: Before modifying or reordering factor levels, make sure that the new levels are consistent with the original ones. Use the
levels()
function to inspect the current levels.
Tip: Always inspect your factor levels after subsetting or modifying your data to ensure they are aligned with your expectations.
Example of Correct Factor Level Management
Original Factor | Modified Factor |
---|---|
Level Order: Low, Medium, High | Level Order: Low, Medium, High |
Unused Level: Very High | Unused Level Dropped |